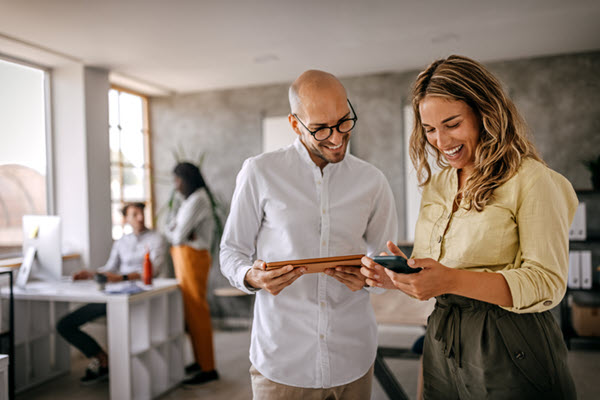
AngularJS is a widely adopted JavaScript framework, arming developers with a rich arsenal of tools to engineer dynamic and captivating web applications. Notably, its robust routing capabilities emerge as a key pillar for constructing Single Page Applications (SPAs). Effectively orchestrating navigation and dynamically presenting diverse content sans the need for complete page refreshes, AngularJS routing emerges as a cornerstone in contemporary web development. In this comprehensive guide, we will embark on a detailed exploration of AngularJS routing, meticulously dissecting its foundational principles. Moreover, we will equip you with tangible instances to foster a more profound comprehension and proficiency in navigating this vital element. Nevertheless, before delving into the complexities of AngularJS routing, it’s crucial to take a moment to explore the benefits inherent in Single Page Applications (SPAs). Then, we’ll explore how to implement both an about route as dynamic content and a product route as a dynamic route with practical code examples.
Benefits of Building SPAs with AngularJS Routing
Building SPAs with AngularJS brings a myriad of benefits to both developers and end-users. Some of these include:
Enhanced User Experience
By requesting only necessary information and resources from the server, Single-Page Applications (SPAs) maximize performance by reducing latency and enhancing overall speed and efficiency. Our optimized Method guarantees quick loading times and improves user experience by prioritizing relevant content delivery.
Improved Performance
SPAs fetch just the vital records and assets from the server, reducing latency and enhancing performance.
Simplified Development
AngularJS streamlines development by supplying a modular, element-based design that makes large-scale applications easier to manipulate and preserve.
Cross-Platform Compatibility
SPAs built with AngularJS are inherently cell-pleasant and can be easily adapted for diverse devices and display sizes.
Now that we’ve covered the blessings of SPAs, let’s investigate AngularJS routing and how it improves dynamic and attractive internet apps.
Understanding AngularJS Routing
AngularJS routing is based on the concept of mapping URLs to different views or templates within the application. It enables developers to define routes and associate each route with a specific template and controller. When a user navigates to a particular URL, AngularJS loads the associated template and controller, updating the view dynamically without requiring a full page reload.
Understanding Dynamic Content
Dynamic content refers to website elements or data that can change dynamically without requiring a full page reload. This dynamism enables developers to create more engaging and personalized user experiences by updating content in real-time based on user interactions or other factors. In AngularJS, dynamic content is typically achieved through data binding and the manipulation of model data.
Understanding Dynamic Routes
However, dynamic routes allow developers to outline routes with dynamic parameters that could be exchanged primarily based on person entry or other situations. These dynamic parameters act as placeholders in the route path, capturing values from the URL and allowing for the dynamic rendering of content. Dynamic routes provide a flexible and powerful mechanism for handling different types of content within an application.
Key Concepts
1. ngRoute
ngRoute is a module provided by AngularJS that enables routing capabilities in an application. Including this module as a dependency when defining an AngularJS application that utilizes routing is essential.
2. $routeProvider
The $routeProvider service is a crucial component of critical routing. It allows developers to configure routes within an application by defining URL paths and associating them with specific templates and controllers.
3. ng-view Directive
The ng-view
directive plays a crucial role in AngularJS, indicating precisely where within an HTML template AngularJS should inject the contents of the current route’s template. It is a placeholder for rendering dynamic perspectives based on the cutting-edge route.
4. Controllers
Controllers in AngularJS are JavaScript functions that are responsible for defining the behavior and data associated with a particular view. Controllers play a crucial role in separating concerns within an application by handling view logic and interactions.
5. Template
In AngularJS routing, a template denotes an HTML file representing a distinct view within the application. These templates are linked with routes and dynamically loaded and rendered based on the current accessed route.
6. otherwise Method
The otherwise() Method specifies a default route to navigate if the requested route doesn’t match any of the defined routes. It ensures users are redirected to a designated route when accessing unrecognized URLs.
Example AngularJS Routing Implementation
Let’s dive into a practical example to demonstrate AngularJS routing in action.
Setting Up AngularJS Routing
To use routing in an AngularJS application, you first need to include the AngularJS and ngRoute libraries for your undertaking (index.html). You can download them from the official website or include them via a CDN link in your HTML file.
<script src=" <script src="
You can also leverage package managers like NPM to manage your project dependencies more efficiently.
npm install angular angular-route
By going for walks with the above command, you may set up AngularJS and the angular-path module, which offers routing competencies, as dependencies in your project.
Once you’ve included AngularJS, you can define your application module and configure routing using the $routeProvider
service provided by AngularJS.
routeProvider.js
var app = angular.module("myApp", ["ngRoute"]); app.config(function ($routeProvider, $locationProvider) { $locationProvider.hashPrefix(""); $routeProvider .when("/", { templateUrl: "views/home.html", controller: "HomeController", }) .when("/about", { templateUrl: "views/about.html", controller: "AboutController", }) .when("/product/:id", { templateUrl: "views/product.html", controller: "ProductController", }) .otherwise({ redirectTo: "/" }); }); app.controller("MainController", function ($scope) { // MainController logic here });
In the above code:
- We define the application module `myApp` and specify the dependency on the `ngRoute` module, which provides routing capabilities.
- We configure routes using the `$routeProvider.when()` Method. Each route definition consists of a URL path, a template URL, and, optionally, a controller.
- We’ve added the $locationProvider.hashPrefix(”) configuration to remove the default hashbang (#!) from the URL.
- The `otherwise()` Method specifies the default route to navigate if the requested route doesn’t match any defined routes.
Creating Views and Controllers
Now that we’ve configured routing let’s create the views and controllers for our routes.
Home View and Controller
Create a file named home.html
inside a directory called views.
This will be the template for the home route.
home.html
<div ng-controller="HomeController"> <h1>Welcome to the Home Page</h1> <p>This is the home page of our AngularJS application.</p> </div>
Next, create a controller named HomeController
in the routeProvider.js file.
routeProvider.js
app.controller("HomeController", function ($scope) { //Home Controller logic here });
Implementing Dynamic Content and Routes
Let’s now see how we can implement both an about route as dynamic content and a product route as a dynamic route in an AngularJS application.
About View and Controller as Dynamic Content
Let’s observe how we can enforce dynamic content in AngularJS. Similarly, create a report named about.html
in the perspectives
listing.
about.html
<div ng-controller="AboutController"> <h1>{{ pageTitle }}</h1> <p>{{ pageContent }}</p> </div>
In this about.html template:
- We use AngularJS expressions ({{ }}) to dynamically render the page title and content, which are bound to the corresponding scope variables (pageTitle and pageContent) set in the AboutController.
- When navigating the About page, users will see the dynamically populated title and content.
In the AboutController, we can dynamically set the page title and content based on route parameters or any other logic specific to the about page. Then, define the <strong>AboutController</strong>
by adding the mentioned code in the routeProvider.js file.
routeProvider.js
app.controller("AboutController", function ($scope) { $scope.pageTitle = "About Us"; $scope.pageContent = "Learn more about our company and mission here."; });
Product View and Controller as Dynamic Route
Create the product view template (product.html) and define the corresponding controller (ProductController) to handle the dynamic product data.
product.html
<div ng-controller="ProductController"> <h1>Product Details</h1> <p>ID: {{ productId }}</p> </div>
In the above product.html template, we display the product details, including the product ID obtained from the $routeParams service.
Then, define the ‘ProductController’ by adding the mentioned code in the routeProvider.js file.
routeProvider.js
app.controller('ProductController', function($scope, $routeParams) { $scope.productId = $routeParams.id; // Fetch product data based on the ID and update the view });
The ProductController extracts the product ID from the route parameters and can then use it to fetch the corresponding product data from the server or another data source.
Linking Routes to Navigation
For seamless navigation between various routes, leverage the ng-href
directive within your HTML to establish links to different paths specified in your route definitions.
index.html
<div ng-controller="MainController"> <ul> <li><a ng-href="#/">Home</a></li> <li><a ng-href="#/about">About</a></li> <li><a ng-href="#/product/1">Product 1</a></li> <li><a ng-href="#/product/2">Product 2</a></li> </ul> <div ng-view></div> </div>
Note: When implementing AngularJS routing, remember to add the ng-app= “myApp” attribute to the opening <html> tag in your index.html file. Additionally, link the routeProvider.js file in your index.html to enable routing functionality.
The ng-view
directive is a placeholder where AngularJS will inject the templates associated with the current route.
Output
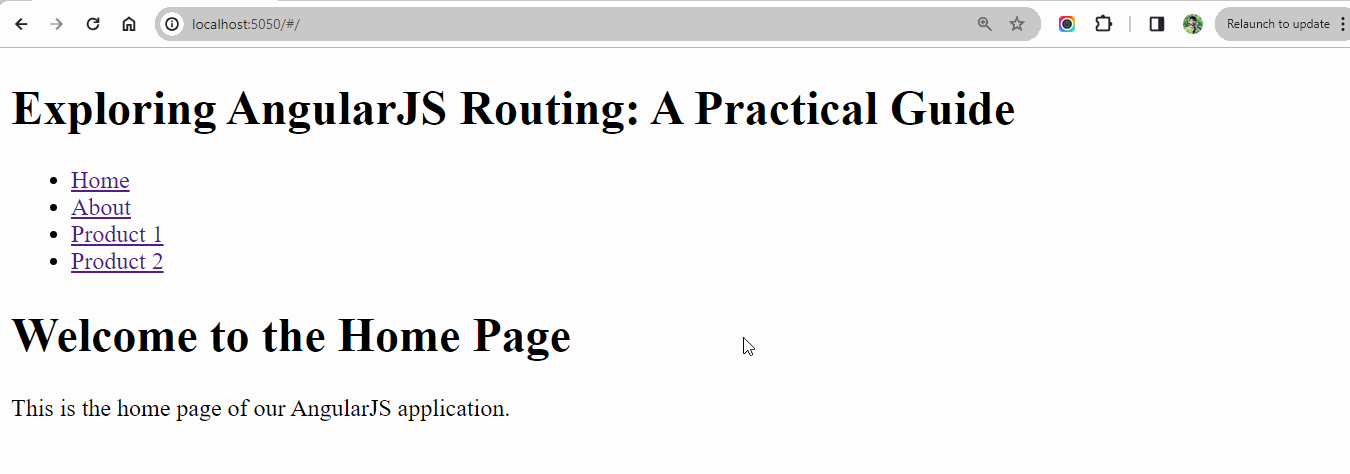
Conclusion
AngularJS routing provides a powerful mechanism for building SPAs by enabling navigation between different views without page reloads. Developers can create dynamic and interactive web applications by configuring routes, defining templates, and linking them to controllers. With the examples and explanations provided in this guide, you should now understand AngularJS routing and be well-equipped to leverage it in your projects.
Remember to explore further documentation and best practices to enhance your routing implementation and create seamless user experiences in your AngularJS applications. Happy coding!