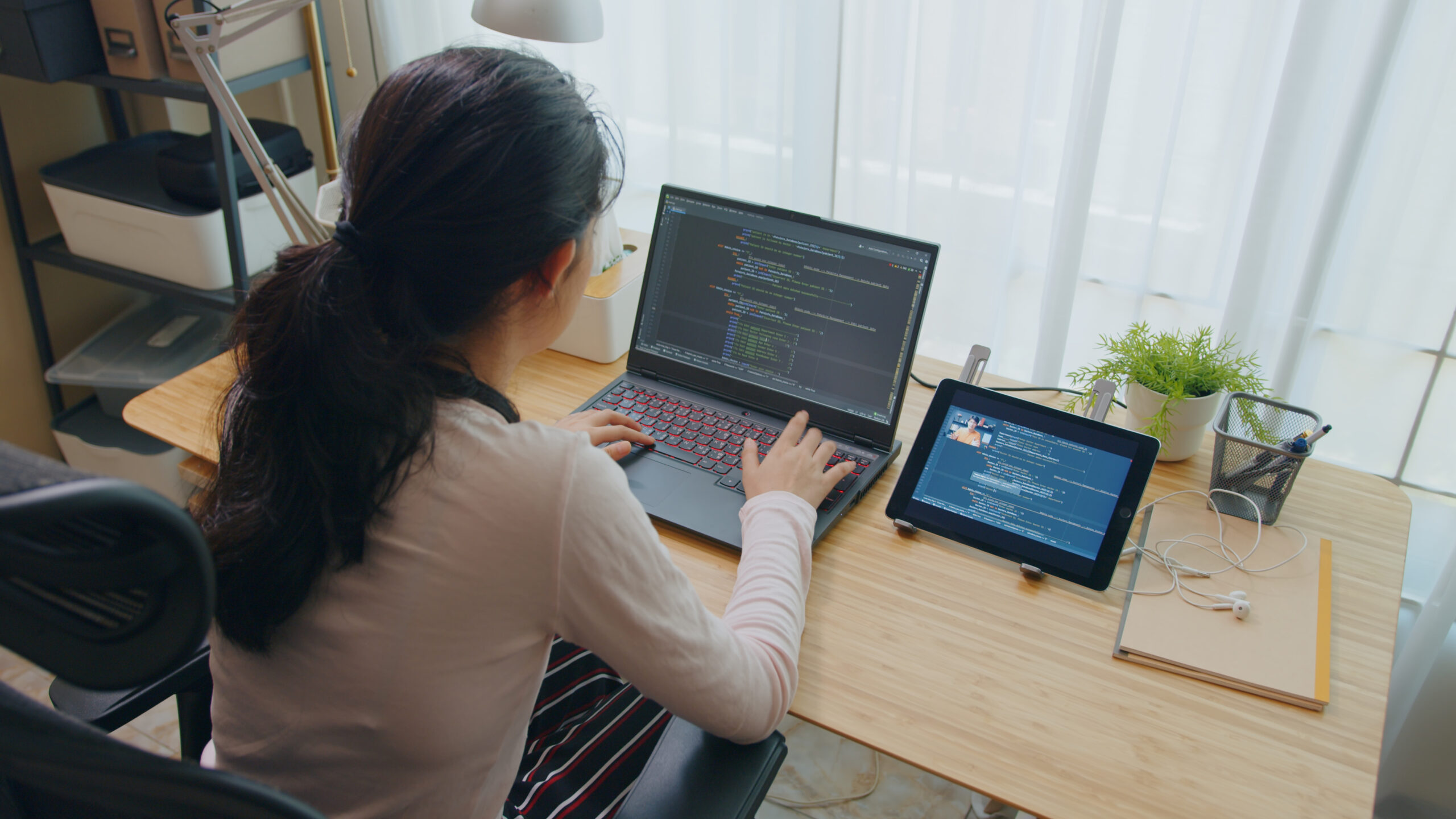
Welcome back to the second installment of our three-part series on Cypress, the premier tool for testing web applications. Having covered the basics in our first article, we now turn our focus to advanced testing techniques that can further enhance your testing strategy. This article aims to explore the depths of Cypress’s capabilities, helping you leverage its full potential for more complex testing scenarios.
User Interaction Testing
Interacting with web elements is a cornerstone of end-to-end testing, and Cypress excels in simulating real user behaviors. Here’s how you can test various user interactions:
cy.get(‘button’).click(); // – Simulates a button click
cy.get(‘input’).type(‘Hello, Cypress!’); // – Types text into an input field
cy.fixture(“data.cvs”).as(‘myFixture’) // – Loads data.cvs located into fixture folder
cy.dataCy(‘input’).selectFile(‘@myFixture’); // – Sets a file as the value for the file input element.
cy.get(‘form’).submit(); // – Submits a form
These commands allow you to mimic user actions closely, ensuring your application behaves as expected in real-world scenarios.
Mocking and Stubs with Cypress
Mocking API calls and stubbing responses are crucial for isolating and testing components or pages in your application. Cypress provides a straightforward way to intercept and control the behavior of network requests:
cy.intercept(‘GET’, ‘/api/users’, { fixture: ‘users.json’ }).as(‘getUsers’);
cy.wait(‘@getUsers’).its(‘response.statusCode’).should(‘eq’, 200);
This example demonstrates intercepting an API call and using a fixture to mock the response, ensuring your tests are not dependent on external systems.
Custom Commands
Cypress allows you to create custom commands, enhancing the reusability of your code and making your tests cleaner and more maintainable. Here’s how to define a custom command:
Cypress.Commands.add(‘login’, (email, password) => {
cy.get(‘input[email]’).type(email);
cy.get(‘input[password]’).type(password);
cy.get(‘form’).submit();
});
You can then use this command in any test:
cy.login(‘[email protected]’, ‘password’);
Parallel Testing with Cypress
As your test suite grows, running tests in parallel can significantly reduce your overall testing time. Cypress Dashboard provides an easy way to run tests concurrently across multiple machines, optimizing your CI/CD pipeline’s efficiency.
Best Practices for Advanced Testing
- Organize Tests Logically: Group related tests using describe blocks to keep your test suite organized and readable.
- Use stable selectors: Use data-* attributes to provide context to your selectors and isolate them from CSS or JS changes. Don’t target elements based on CSS attributes such as: id, class, tag. Don’t target elements that may change their textContent. Don’t use too generic selector (e.g. cy.get(button)) Don’t couple it to styles.
- Test unhappy paths:Don’t just test the ‘happy path’ of the user. Make sure to test users that might be maliciously using your app or actions that might not be common.
- Don’t assign return values: Commands are enqueued and run asynchronously. To access what each Cypress command yields you use .then()
- Don’t test external sites: Only test websites that you control. Try to avoid visiting or requiring a 3rd party server. If you choose, you may use cy.request() to talk to 3rd party servers via their APIs. If possible, cache results via cy.session() to avoid repeat visits
- Keep tests independent: Don’t make one test dependent on another. This becomes extremely difficult to manage.
- Don’t write tiny tests: Writing tiny tests, like unit tests, is non-performant and excessive. Cypress resets various states and tests between tests that takes a long time. So small tests hurt performance. Plus, you’ll still know exactly what assertion fails in a longer e2e test.
- Clen up state before tests run: Don’t clean up state with after or afterEach. One benefit of Cypress is incrementally writing tests and application code. And if the state isn’t maintained after a test, it can make it more difficult to know what you should test next. If something fails in the middle of your test, the after cleanup functions won’t get a chance to run. Cypress already cleans up state between tests, so this might not be something you need to worry about at all.
- Using unnecessary waiting: Don’t clean up state with after or afterEach. One benefit of Cypress is incrementally writing tests and application code. And if the state isn’t maintained after a test, it can make it more difficult to know what you should test next. If something fails in the middle of your test, the after cleanup functions won’t get a chance to run. Cypress already cleans up state between tests, so this might not be something you need to worry about at all.
- Continuous Integration: Integrate Cypress with your CI/CD pipeline to ensure tests are automatically run at key stages of development.
Conclusion
Mastering advanced testing techniques with Cypress not only enhances the quality and reliability of your web applications but also streamlines your development workflow. By leveraging user interaction testing, mocking and stubs, custom commands, and parallel testing, you can build a robust testing strategy that scales with your project.
Stay tuned for Part 3 of our series, where we will explore integrating Cypress into your development workflow for continuous testing excellence.