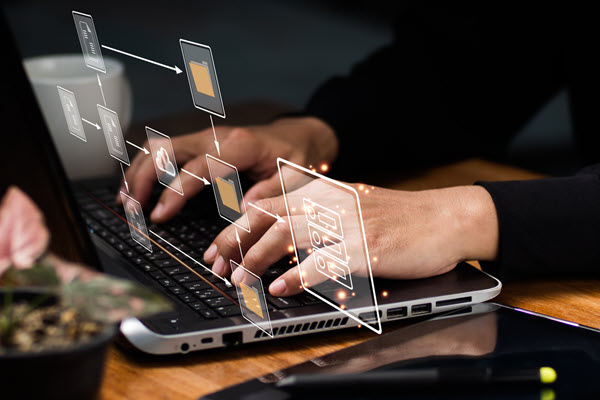
Welcome back to the continuation of our journey through crucial Web APIs! For newcomers to our series, I highly recommend diving into Part 1, accessible here, where we extensively cover the fundamental aspects of the Web Forms API. Continuing our journey, let’s delve into further exploration of vital Web APIs, including the Web History API and Web Storage API. With the Web History API enabling effortless navigation through browsing history and the Web Storage API providing flexible storage solutions via local storage and session storage, we’re set to uncover their functionalities in detail.
Web History API
The Web History API encompasses methods and properties that enable JavaScript to interact with the browser’s history stack. It equips developers with tools to navigate user browsing history, manage session history, and execute operations pertinent to page navigation.
Let’s explore some of the key functions and properties along with code examples:
The Web History API encompasses methods and properties that enable JavaScript to interact with the browser’s history stack. It equips developers with tools to navigate user browsing history, manage session history, and execute operations pertinent to page navigation.
In essence, history.go() is a crucial function within the Web History API, empowering users to traverse their browsing history programmatically. This method, accepting positive or negative integers as arguments, facilitates navigation forward or backward by the designated number of pages, mirroring the functionality of the browser’s navigation controls.
Put plainly, history.forward() is a function in the Web History API permitting users to advance to the subsequent page in their browsing history. It mimics the action of clicking the forward button in the browser, facilitating seamless navigation through visited pages.
In simple terms, history.length is a property in the Web History API representing the number of entries in the browsing history stack. Each entry corresponds to a unique URL visited by the user during the current browsing session.
Here’s a simple example demonstrating the usage of Web History API key functions and properties:
HTML:
<button onclick="goBack()">Go Back</button> <button onclick="goFunction()">Go Function</button> <button onclick="checkHistoryLength()">Check History Length</button> <button onclick="goForward()">Go Forward</button>
JavaScript:
function goBack() { window.history.back(); } function goFunction() { window.history.go(1); // Go forward one page } function checkHistoryLength() { let length = window.history.length; console.log("Number of pages in history:", length); } function goForward() { window.history.forward(); }
In the JavaScript code, four functions are defined to interact with the Web History API:
- goBack(): When the associated button clicks, it calls history.back(), navigating to the previous page in the browsing history.
- goForward(): When the associated button is clicked, it calls history.forward(), navigating to the next page in the browsing history.
- goToPage(): When the associated button is clicked, it calls history.go(2), navigating two pages forward in the browsing history.
- displayHistoryLength(): This function fetches the total count of pages stored in the browsing history stack using ‘window.history.length’ and then outputs this count to the console for reference.
This example demonstrates how JavaScript can be used to create interactive navigation controls that enhance the user experience by leveraging the Web History API.
Web Storage API
The Web Storage API in web browsers enables developers to store data locally. It offers two main mechanisms: localStorage and sessionStorage, allowing storage of key-value pairs.
- localStorage: This approach enables data to be stored without any predefined expiration, ensuring its persistence across browser sessions, including closure and reopening.
- sessionStorage: This mechanism stores data for the duration of the page session. The stored data is cleared when the page session ends, typically when the user closes the browser tab.
Let’s explore some of the methods and properties along with code examples:
setItem():
Put plainly, setItem() is a function within the Web Storage API that permits storing data directly in the user’s browser. It saves data in key-value pairs, each being distinct and linked to a particular value.
Here’s an example demonstrating the usage of setItem():
HTML:
<button onclick="saveData()">Save Data</button>
JavaScript:
function saveData() { localStorage.setItem('username', 'John'); console.log('Data saved successfully.'); }
In the provided JavaScript code, the saveData() function is defined. Upon clicking the designated button, the function is activated. Encapsulated within the script is localStorage.setItem(‘username’, ‘John’), where the value ‘John’ is designated to the local storage key ‘username’. This functionality ensures the secure preservation of user-specific details, such as preferences or settings, guaranteeing their accessibility throughout the browsing session. Leveraging JavaScript’s simplicity, developers seamlessly integrate local storage functionality, enhancing web application user experience.
Output:
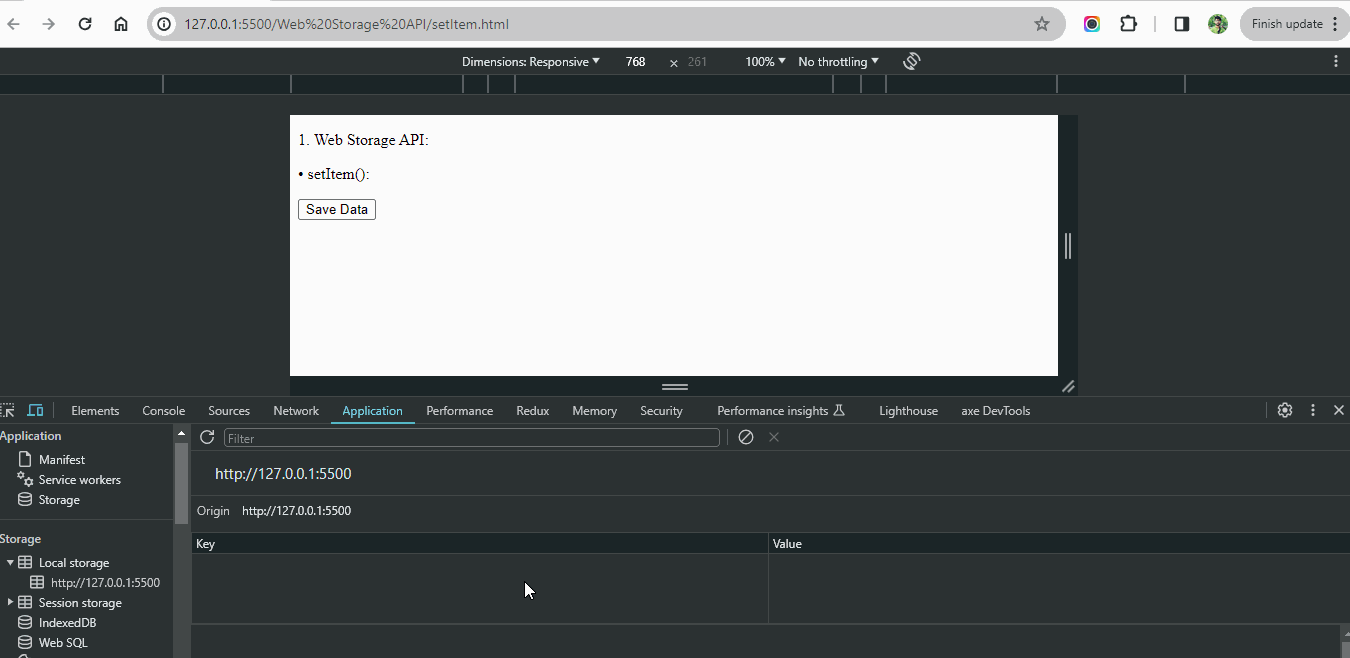
getItem():
Put plainly, getItem() serves as a function within the Web Storage API, enabling the retrieval of locally stored data in the user’s browser. This method grants access to the value linked to a particular key set earlier via the setItem() method.
Here’s a simple example demonstrating the usage of getItem():
HTML:
<button onclick="retrieveData()">Retrieve Data</button>
JavaScript:
function retrieveData() { let username = localStorage.getItem('username'); console.log('Username:', username); }
In the provided JavaScript code, the retrieveData() function is defined. Upon activation of the designated button, the function is initiated. Within its confines, the script localStorage.getItem(‘username’) retrieves the value linked to the ‘username’ key from the browser’s local storage. This retrieved value is subsequently allocated to the variable username, facilitating access and display of the stored data. JavaScript’s adaptability and straightforwardness make it indispensable in web development, empowering developers to craft resilient and feature-laden web applications with local storage capabilities.
Output:
key(n):
Put plainly, key(n) is a function within the Web Storage API permitting the retrieval of the name of the key housed at a designated index position in the storage. This functionality facilitates sequential access to key names, traversing through all keys stored within the browser’s storage system.
Here’s a simple example demonstrating the usage of key(n):
HTML:
<button onclick="displayKey()">Display Key</button>
JavaScript:
function displayKey() { let keyName = localStorage.key(0); console.log("First key:", keyName); }
In the provided JavaScript code, the displayKey() function is defined. Upon clicking the related button, this function activates. Within the function scope, localStorage.key(0) fetches the identifier of the key stored at index 0 within the browser’s local storage. Subsequently, this retrieved key is assigned to the variable keyName, facilitating its utilization for accessing and presenting the respective key. This technique permits iteration through all the keys stored in the local storage, facilitating actions tailored to each. JavaScript’s adaptability and straightforwardness establish it as a cornerstone in web development, empowering developers to craft dynamic and engaging web applications.
Output:
length:
Length is a property within the Web Storage API that furnishes the count of key-value pairs housed in the browser’s storage. It furnishes a means to ascertain the overall count of items in storage, facilitating iteration or other actions reliant on the storage’s magnitude.
For instance, consider this straightforward illustration showcasing the application of length:
HTML:
<button onclick="displayLength()">Display Length</button>
JavaScript:
function displayLength() { let totalItems = localStorage.length; console.log('Total items:', totalItems); }
In the provided JavaScript code, the displayLength() function is defined. Upon clicking the designated button, activation of this function ensues. Within its confines, localStorage.length retrieves the collective count of key-value pairs archived in the browser’s local storage. This resultant value is then allocated to the variable total items, facilitating the assessment of storage magnitude. JavaScript’s inherent ease of use and adaptability make it an indispensable ally in the realm of web development, empowering creators to fashion vibrant and immersive web experiences complete with features like local storage.
Output:
removeItem(keyname):
Put plainly, removeItem() is a function within the Web Storage API that serves to eliminate a designated key-value pair from the browser’s storage. It operates by accepting the key name as input and subsequently erasing the associated value, thus effectively expunging the item from the storage repository.
Here’s a simple example demonstrating the usage of removeItem(keyname):
HTML:
<button onclick="removeData()">Remove Data</button>
JavaScript:
function removeData() { localStorage.removeItem("username"); console.log("Data removed successfully."); }
In the provided JavaScript code, the removeItem() function is defined. Upon clicking the designated button, activation of this function ensues. Within its scope, the script localStorage.removeItem(‘username’) undertakes the removal of the key-value pair bearing the identifier ‘username’ from the browser’s local storage. This action effectively removes the item, allowing you to clean up unnecessary data stored in the browser. JavaScript’s simplicity and versatility make it a fundamental tool for web development, enabling developers to create robust and feature-rich web applications with functionalities like local storage manipulation.
Output:
clear():
Put plainly, clear() is a method within the Web Storage API that enables the wholesale removal of all key-value pairs housed within the browser’s storage mechanism. It effectively clears the entire storage, removing all data stored within it.
Here’s a simple example demonstrating the usage of clear():
HTML:
<button onclick="clearStorage()">Clear Storage</button>
JavaScript:
function clearStorage() { localStorage.clear(); console.log('Storage cleared successfully.'); }
In the provided JavaScript code, the clearStorage() function is defined. When the associated button is clicked, this function is triggered. Inside the function, localStorage.clear() removes all key-value pairs from the browser’s local storage. This action effectively clears the entire storage, removing all data stored within it. JavaScript’s versatility and ease of use position it as an essential asset in web development, granting developers the capability to effectively oversee browser storage for diverse applications, including caching, session control, and user preferences management.
Output:
Conclusion:
In this part, we’ve explored the foundational aspects of JavaScript Web APIs, focusing on the Web History API for navigation control and the Web Storage API for local data storage. These APIs are essential tools for building robust and interactive web applications. Stay tuned for Part 3 where we’ll explore additional crucial Web APIs. Click here to read Part 3.
Keep exploring and innovating with JavaScript!