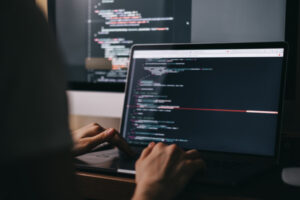
In continuation of Part 1, we will now delve into essential array methods that enhance your JavaScript toolkit. These methods allow you to effortlessly handle complex data manipulation tasks, opening new possibilities for your projects.
isArray()
isArray() is your trusty detector for identifying arrays. Just pass any value to Array.isArray(), and it quickly tells you whether it’s an array or not. Simple, yet incredibly useful for handling different types of data.
Example:
const animals = ["Cheetah", "Lion", "Zebra", "Horse", "Deer"]; const isArray = Array.isArray(animals); console.log("Is passed value an array: ", isArray);
Output:

keys(), values(), and entries()
These methods give you iterators for keys, values, and key-value pairs within an array. Essentially, they provide different ways for you to move through the elements of an array.
Key
const fruits = ["apple", "banana", "orange"]; for (const key of fruits.keys()) { console.log("Keys of an array: ", key); }
Output:
value
const fruits = ["apple", "banana", "orange"]; for (const value of fruits.values()) { console.log("Values of an array: ", value); }
Output:
entries
const fruits = ["apple", "banana", "orange"]; for (const [index, fruit] of fruits.entries()) { console.log("Values of array with its index: ", index, fruit); }
Output:
from()
Array.from(): It’s like a magic wand that turns almost anything into an array. Whether you have scattered pieces of data or an iterable object, Array.from() neatly organizes them into a new array, ready for action.
Example:
const arrayLike = { length: 3, 0: "a", 1: "b", 2: "c" }; const newArray = Array.from(arrayLike); console.log(newArray);
Output:
fill()
The fill() method is used to change all elements in an array to a static value.
Example:
const numbers = [1, 2, 3, 4, 5]; numbers.fill(0, 2, 4); // [1, 2, 0, 0, 5] console.log(numbers)
Output:
flat()
Imagine you have a stack of nested trays, each containing some items. flat() is like taking out all the items from those trays and putting them into a single tray, simplifying the organization.
Example:
const nestedArray = [["Peter Pan", "Aladdin"], ["Mulan", "Maleficent"], ["Moana", "Tangled"]]; const flattenedArray = nestedArray.flat(); console.log("flat method:\n",flattenedArray);
Output:
with()
The with() method is a tool for arrays that lets you change one specific item without touching the original array. It creates a new array reflecting the update.
Syntax
newArray = array.with(index, newValue);
Parameters
- index: This represents the position of the item that needs to be updated.
- newValue: The new value for the specified item.
Example:
const originalArray = ['apple', 'banana', 'cherry']; const newArray = originalArray.with(1, 'grape'); console.log("New Array:",newArray); console.log("Orignal Array:",originalArray);
Output:
length
Length tells you how many items are in an array or characters in a string.
Example:
const languages = ["Urdu", "English", "Hindi", "Spanish", "Italian"]; console.log("Length of array:",languages.length);
Output:
at()
Get the item located at a particular position within an array or a string, considering positive and negative indexes.
Example:
const languages = ["Urdu", "English", "Hindi", "Spanish", "Italian"]; console.log("Element at end of the array:",languages.at(-1));
Output:
join()
The function combines all elements of an array into a single string, separating them with a specified delimiter.
Example:
const series = ["Array", "Methods", "In", "JavaScript"]; console.log("Series:",series.join(' '));
Output:
delete()
Removes a property from an object; it is not typically used for arrays as it leaves undefined holes.
Example:
const languages = ["Urdu", "English", "Hindi", "Spanish", "Italian"]; delete languages[1]; console.log("After deleting element at 1",languages);
Output:
spread (…)
This method enables an array or other iterable object to be spread out in locations where functions or arrays anticipate zero or more arguments or elements.
Example:
const languagesOne = ["Urdu", "English"]; const languagesTwo = ["Spanish", "Italian"]; const allLanguages = [...languagesOne, ...languagesTwo]; // Combines languagesOne and languagesTwo into allLanguages console.log("Combined array:",allLanguages);
Output:
toString()
converts all elements of an array into a single string, separating them with commas.
Example:
const languages = ["Urdu", "English", "Hindi", "Spanish", "Italian"]; let languagesString = languages.toString(); console.log("Array to String:",languagesString);
Output:
Conclusion:
As we wrap up the essential and primary part of our exploration into JavaScript array methods, remember that these foundational techniques serve as the building blocks for more complex operations. Mastery of adding, removing, and manipulating elements within arrays opens a myriad of possibilities for data handling in your JavaScript projects. Stay tuned for the next part of our series, where we’ll delve into advanced array methods and further expand our JavaScript toolkit.