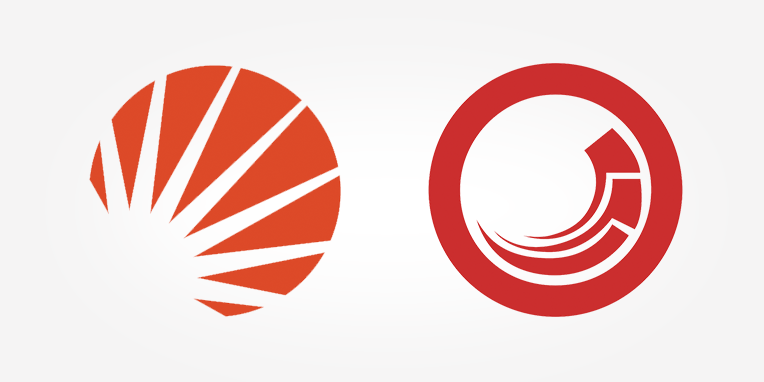
Apache Solr provides several functionalities, and recently, I have used the auto-suggest and spell-check functionality in my project. The primary requirement was to integrate functionality that offers suggestions on the search term. For example, a text box enabled the suggester component, which displays the list of suggestions as the user types a query term in the text box, and the user can select from the list to complete the search term. You can also use the spell check feature and the suggester component to auto-correct the search term. To use these APIs, we require Sitecore 9 or later.
In my project, a few drop-down components were implemented in React JS, and others used the SXA OOTB Search component. If we use the SXA OOTB component, it uses SXA indexes where we don’t require any change. We can set up everything through Sitecore itself.
First, let’s enable the feature in Solr for the custom search component,
Solr Suggest (Autocomplete functionality)
Configure a suggester component in Solr
To enable the suggester component for the Solr index, open the solrconfig.xml file from the index conf folder. We need to uncomment/add the below line of code.
<!--Start: Search component: Solr Suggester--> <searchComponent name="suggest" class="solr.SuggestComponent"> <lst name="suggester"> <str name="name">Suggester Name</str> <str name="lookupImpl">Select the lookup implementation</str> <str name="dictionaryImpl">Select the dictionary implementation</str> <str name="field">add filed name</str> <str name="suggestAnalyzerFieldType">string/text_general</str> <str name="buildOnStartup">false</str> <str name="buildOnCommit">false</str> <str name="exactMatchFirst">true</str> </lst> </searchComponent> <!--End: Search component: Solr Suggester-->
Two things need to be configured correctly in the above configurations,
- Dictionary Implementation Parameter
- Lookup Implementation Parameter
Dictionary Implementation
We need to configure this in the “dictionaryImpl” parameter in the above code snippet. We can use the below implementation for different purposes. Also, you can select multiple dictionaries in one suggester search component. For more information, you can refer to Solr documentation.
DocumentDictionaryFactory – It takes terms, weights, and indexed optional payload.
DocumentExpressionDictionaryFactory – It’s the same as above, “DocumentDictionaryFactory,” but here, the user can specify the expression in the “weightExpression” tag for a more refined response.
HighFrequencyDictionaryFactory – If we use this, then it lists out the most searched and popular terms and removes the less frequent terms.
FileDictionaryFactory – Use this only to show results/suggestions from the external file.
Lookup Implementation
We need to configure this under the “lookupImpl” parameter in the above code snippet. There are multiple implementations available that apply to specific scenarios. I have tried only the below implementation in my project, but many options can provide solutions. To refer to other implementations, refer to this link.
AnalyzingLookupFactory – If you used this implementation, then it first analyzes the search text and hods the suggestions in the weighted FST-in memory data.
FuzzyLookupFactory – It’s the same as the “AnalyzingLookupFactory” implementation; also, it has spell-check functionality enabled by default.
AnalyzingInfixLookupFactory – This implementation analyses the search text and provides a list of suggestions based on prefix matches to any indexed term/text/field.
FSTLookupFactory – It’s an automation-based lookup implementation, and here, they always hold all the suggestions in the FST, but Solr recommends there only if you have highly developer-matching results.
TSTLookupFactory – It’s a ternary-based representation.
Configure the Request Handler for the above search component
To enable the request handler for the above suggester component for the Solr index, open the solrconfig.xml file from the index conf folder. We need to uncomment/add/update the below line of code, and to learn more about the parameters used in the above request handler, refer to this link.
<requestHandler name="/suggest" class="solr.SearchHandler" startup="lazy"> <lst name="defaults"> <str name="suggest">true</str> <str name="suggest.count">response count</str> </lst> <arr name="components"> <str>suggest</str> </arr> </requestHandler>
To use the above features in the backend, we can use the API, which is an extension of the existing Sitecore ContentSearch API, and follow the below steps to use the required API in your project.
Step 1: Installed the below list of DLLs from the NuGet manager,
- SolrNet.dll
- Sitecore.ContentSearch.dll
- Sitecore.ContentSearch.SolrProvider.dll
- Sitecore.ContentSearch.SolrNetExtension.dll
Step 2: Import the below namespaces,
- Sitecore.ContentSearch.SolrNetExtension
- Sitecore.ContentSearch.SolrProvider.SolrNetIntegration
Step 3: Now, you can use the Suggest API in your repository. Create an object of type SolrSuggestQuery, assign the search/request terms in that object, and call the suggest function on the selected index to receive the response in SolrSuggestHandlerQueryResults, which consists of all the suggestions we get as per the search text/query. Refer to the below line of code,
public static IEnumerable<string> GetListOfSuggestions(string SearchTerm) { using(var index = ContentSearchManager.GetIndex("Add-the-name-of-index-here").CreateSearchContext()) { SolrSuggestQuery query = term; var options = new SuggestHandlerQueryOptions { Parameters = new SuggestParameters { Count = 3; Build = true; } }; var result = index.Suggest(query, options); return result.Suggestions[<MySuggester>].Suggestions.Select(a=>a.Term); } }
Note: MySuggester is the name of the suggestion component that we added in the Search Component under the Solr configuration.
Solr Spell-check
Configure a spell-check component in Solr
To enable the spell-check component, we need to uncomment/add the below line of code, which is available in solrconfig.xml of all Solr indexes.
<!-- Spell Check - The spell check component can return a list of alternative spelling suggestions. --> <searchComponent name="spellcheck" class="solr.SpellCheckComponent"> <str name="queryAnalyzerFieldType">text_general</str> <!-- Multiple "Spell Checkers" can be declared and used by this component --> <!-- a spellchecker built from a field of the main index --> <lst name="spellchecker"> <str name="name">default</str> <str name="field">_text_</str> <str name="classname">solr.DirectSolrSpellChecker</str> <!-- the spellcheck distance measure used, the default is the internal levenshtein --> <str name="distanceMeasure">internal</str> <!-- minimum accuracy needed to be considered a valid spellcheck suggestion --> <float name="accuracy">0.5</float> <!-- the maximum #edits we consider when enumerating terms: can be 1 or 2 --> <int name="maxEdits">2</int> <!-- the minimum shared prefix when enumerating terms --> <int name="minPrefix">1</int> <!-- maximum number of inspections per result. --> <int name="maxInspections">5</int> <!-- minimum length of a query term to be considered for correction --> <int name="minQueryLength">4</int> <!-- maximum threshold of documents a query term can appear to be considered for correction --> <float name="maxQueryFrequency">0.01</float> <!-- uncomment this to require suggestions to occur in 1% of the documents <float name="thresholdTokenFrequency">.01</float> --> </lst>
Also, uncomment/add the below line of code in the same solrconfig.xml file for the request handler for the spell-check feature.
<requestHandler name="/spell" class="solr.SearchHandler" startup="lazy"> <lst name="defaults"> <!-- Solr will use suggestions from both the 'default' spellchecker and from the 'wordbreak' spellchecker and combine them. Collations (re-written queries) can include a combination of corrections from both spellcheckers --> <str name="spellcheck.dictionary">default</str> <str name="spellcheck">on</str> <str name="spellcheck.extendedResults">true</str> <str name="spellcheck.count">10</str> <str name="spellcheck.alternativeTermCount">5</str> <str name="spellcheck.maxResultsForSuggest">5</str> <str name="spellcheck.collate">true</str> <str name="spellcheck.collateExtendedResults">true</str> <str name="spellcheck.maxCollationTries">10</str> <str name="spellcheck.maxCollations">5</str> </lst> <arr name="last-components"> <str>spellcheck</str> </arr> </requestHandler>
For capturing the spell-check result, we need to install the same NuGet packages that we installed for the above Suggest component. We can use SolrNetProxy.GetSpellcheck to get the list of suggestions for spell-check API.
Enable autocomplete feature in Sitecore SXA OOTB Search component
If you are using Sitecore SXA search OOTB Component, then to enable the suggestions, we need to follow the below steps to enable the prediction, and it uses SXA indexes in the background to generate the search suggestions. Refer to the below screenshots with these steps,
Step 1: Set the Search Scope, which helps to set the scope of the search in the content tree.
Step 2: Set up the number of suggestions that we need to show in the drop-down in the Max predictive result count.
Step 3: You can set the language versions as well under the Default language filtering.
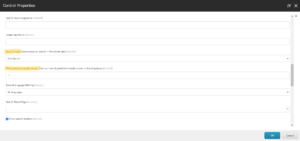
I hope this blog post helps you to implement the auto-suggestion and spell-check functionality using Solr. Feel free to add a comment below if you have any questions or comments.
Happy Learning!