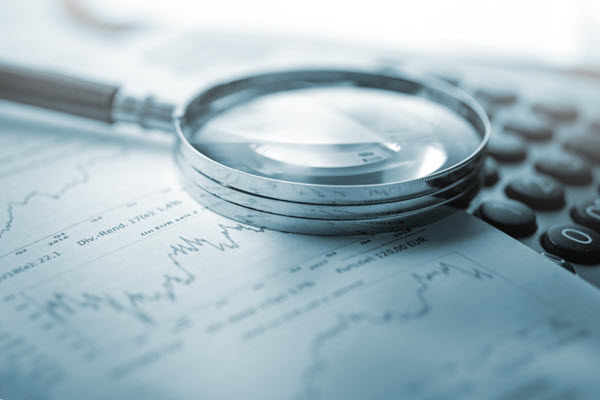
In React development, ensuring proper data validation is crucial for building robust and reliable applications. One powerful tool for type-checking is PropTypes, a built-in feature in React that enables developers to validate the props passed to components. In this blog, we will explore the concept of type-checking with PropTypes, understand its benefits, and provide brief examples to demonstrate its usage.
Why Type-checking with PropTypes Matters:
Type-checking is a fundamental practice that helps catch errors and prevent unexpected behaviour in React components. By defining the expected types of props, PropTypes allows developers to validate and enforce data integrity throughout the application. This not only improves code quality but also enhances the debugging process by providing clear error messages when incorrect data types are passed.
Getting Started with PropTypes:
To begin using PropTypes, you need to install the prop-types
package, which is already included in most React projects. If it’s not installed, you can easily add it using npm or yarn.
npm install --save prop-types
Basic Type-checking
Let’s consider a simple example of a functional component called Account
that receives two props: name
(string) and age
(number).
import PropTypes from 'prop-types'; const Account= ({ name, age }) => { return ( <div> <h2>{name}</h2> <p>{age} years old</p> </div> ); }; Account.propTypes = { name: PropTypes.string.isRequired, age: PropTypes.number.isRequired, };
In the above code, we import the PropTypes
object from the prop-types
package. By assigning the expected types to the propTypes
property of the component, we ensure that the name
prop is of type string and the age
prop is of type number. The isRequired
flag indicates that these props are mandatory.
Handling Default Prop Values
PropTypes also allows us to specify default values for props, which come in handy when a prop is not required or not provided.
import PropTypes from 'prop-types'; const Button = ({ label, color }) => { return ( <button style={{ backgroundColor: color }}>{label}</button> ); }; Button.propTypes = { label: PropTypes.string.isRequired, color: PropTypes.string, }; Button.defaultProps = { color: 'blue', };
In this example, the Button component expects a label prop of type string, which is marked as required using isRequired in the propTypes definition. The color prop is defined as an optional string. If the color prop is not provided, the defaultProps object sets its default value to ‘blue.’ This means that if the color
prop is not explicitly passed.
Validating Objects and Arrays
PropTypes can also be used to validate objects and arrays, allowing for more complex data type validation.
import PropTypes from 'prop-types'; const Product = ({ name, price, features }) => { return ( <div> <h2>{name}</h2> <p>${price}</p> <ul> {features.map((feature, index) => ( <li key={index}>{feature}</li> ))} </ul> </div> ); }; Product.propTypes = { name: PropTypes.string.isRequired, price: PropTypes.number.isRequired, features: PropTypes.arrayOf(PropTypes.string).isRequired, };
In the Product
component, we validate that the name
prop is a string, the price
prop is a number and the features
prop is an array of strings.
Conclusion:
Using PropTypes for Type-checking in React apps is crucial to maintaining data accuracy, reducing errors, and improving debugging. Developers can validate prop types, enhancing the reliability of the code.